In Bash version 4, associative arrays were introduced, and from that point, they solved my biggest problem with arrays in Bash—indexing. Associative arrays allow you to create key-value pairs, offering a more flexible way to handle data compared to indexed arrays.
In simple terms, you can store and retrieve data using string keys, rather than numeric indices as in traditional indexed arrays.
But before we begin, make sure you are running the bash version 4 or above by checking the bash version:
echo $BASH_VERSION

If you are running bash version 4 or above, you can access the associative array feature.
Using Associative arrays in bash
Before I walk you through the examples of using associative arrays, I would like to mention the key differences between Associative and indexed arrays:
Feature | Indexed Arrays | Associative Arrays |
---|---|---|
Index Type | Numeric (e.g., 0, 1, 2) | String (e.g., “name”, “email”) |
Declaration Syntax | declare -a array_name |
declare -A array_name |
Access Syntax | ${array_name[index]} |
${array_name["key"]} |
Use Case | Sequential or numeric data | Key-value pair data |
Now, let’s take a look at what you are going to learn in this tutorial on using Associative arrays:
- Declaring an Associative array
- Assigning values to an array
- Accessing values of an array
- Iterating over an array’s elements
1. How to declare an Associative array in bash
To declare an associative array in bash, all you have to do is use the declare
command with the -A
flag along with the name of the array as shown here:
declare -A Array_name
For example, if I want to declare an associative array named LHB
, then I would use the following command:
declare -A LHB

2. How to add elements to an Associative array
There are two ways you can add elements to an Associative array: You can either add elements after declaring an array or you can add elements while declaring an array. I will show you both.
Adding elements after declaring an array
This is quite easy and recommended if you are getting started with bash scripting. In this method, you add elements to the already declared array one by one.
To do so, you have to use the following syntax:
my_array[key1]="value1"
In my case, I have assigned two values using two key pairs to the LHB
array:
LHB[name]="Satoshi"
LHB[age]="25"

Adding elements while declaring an array
If you want to add elements while declaring the associative array itself, you can follow the given command syntax:
declare -A my_array=(
[key1]="value1"
[key2]="value2"
[key3]="value3"
)
For example, here, I created a new associated array and added three elements:
declare -A myarray=(
[Name]="Satoshi"
[Age]="25"
[email]="[email protected]"
)
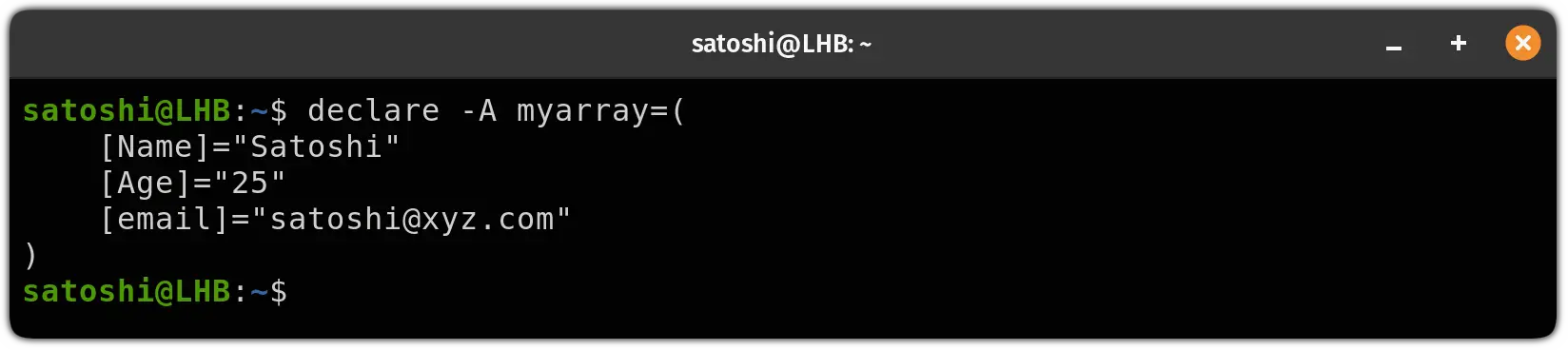
3. Create a read-only Associative array
If you want to create a read-only array (for some reason), you’d have to use the -r
flag while creating an array:
declare -rA my_array=(
[key1]="value1"
[key2]="value2"
[key3]="value3"
)
Here, I created a read-only Associative array named MYarray
:
declare -rA MYarray=(
[City]="Tokyo"
[System]="Ubuntu"
[email]="[email protected]"
)
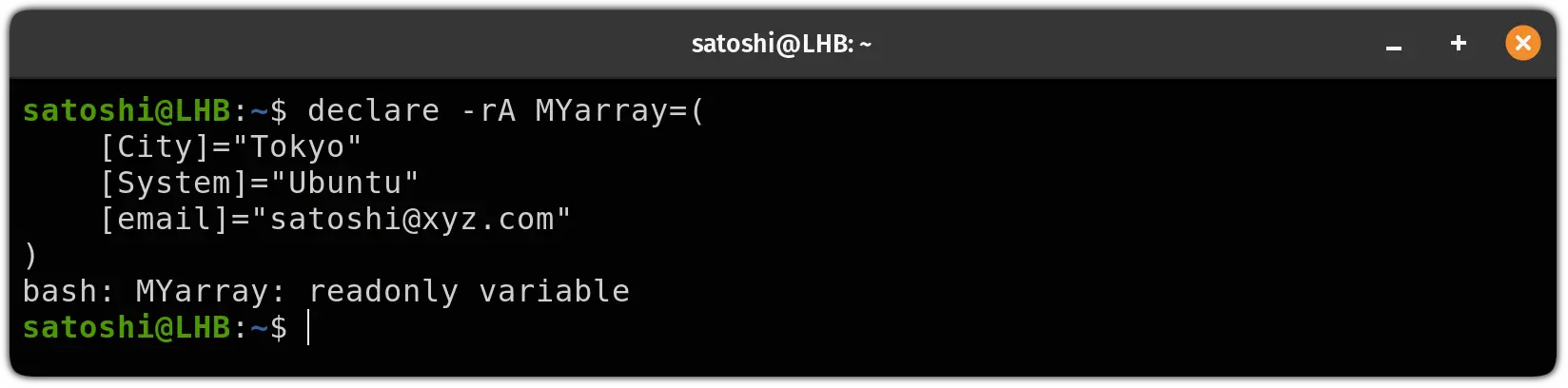
Now, if I try to add a new element to this array, it will throw an error saying “MYarray: read-only variable”:

4. Print keys and values of an Associative array
If you want to print the value of a specific key (similar to printing the value of a specific indexed element), you can simply use the following syntax for that purpose:
echo ${my_array[key1]}
For example, if I want to print the value of email
key from the myarray
array, I would use the following:
echo ${myarray[email]}

Print the value of all keys and elements at once
The method of printing all the keys and elements of an Associative array is mostly the same. To print all keys at once, use ${!my_array[@]}
which will retrieve all the keys in the associative array:
echo "Keys: ${!my_array[@]}"
If I want to print all the keys of myarray
, then I would use the following:
echo "Keys: ${!myarray[@]}"

On the other hand, if you want to print all the values of an Associative array, use ${my_array[@]}
as shown here:
echo "Values: ${my_array[@]}"
To print values of the myarray
, I used the below command:
echo "Values: ${myarray[@]}"

5. Find the Length of the Associative Array
The method for finding the length of the associative array is exactly the same as you do with the indexed arrays. You can use the ${#array_name[@]}
syntax to find this count as shown here:
echo "Length: ${#my_array[@]}"
If I want to find a length of myarray
array, then I would use the following:
echo "Length: ${#myarray[@]}"

6. Iterate over an Associative array
Iterating over an associative array allows you to process each key-value pair. In Bash, you can loop through:
- The keys using
${!array_name[@]}
. - The corresponding values using
${array_name[$key]}
.
This is useful for tasks like displaying data, modifying values, or performing computations. For example, here I wrote a simple for loop to print the keys and elements accordingly:
for key in "${!myarray[@]}"; do
echo "Key: $key, Value: ${myarray[$key]}"
done
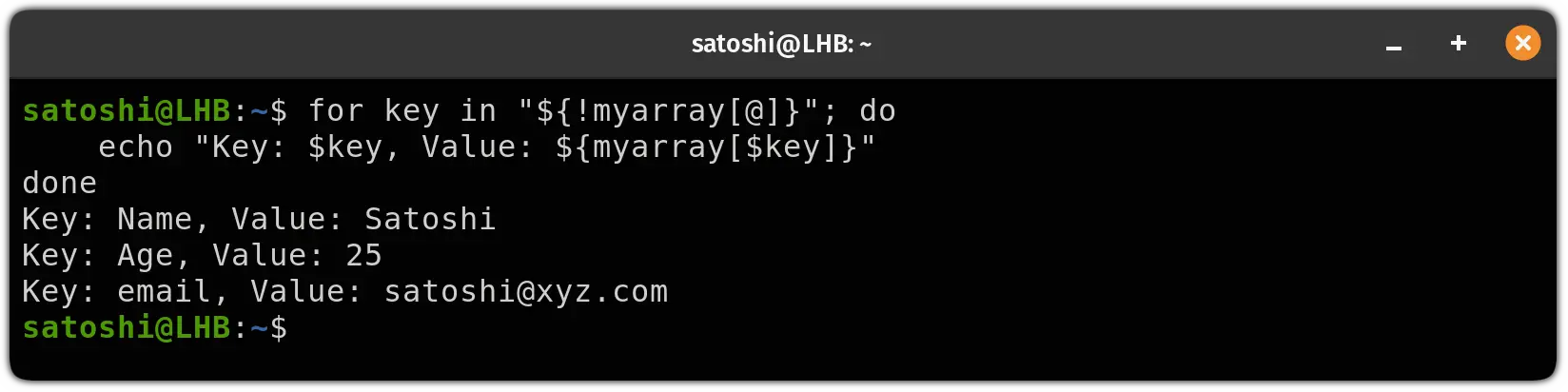
7. Check if a key exists in the Associative array
Sometimes, you need to verify whether a specific key exists in an associative array. Bash provides the -v
operator for this purpose.
Here, I wrote a simple if else script that uses the -v
flag to check if a key exists in the myarray
array:
if [[ -v myarray["username"] ]]; then
echo "Key 'username' exists"
else
echo "Key 'username' does not exist"
fi
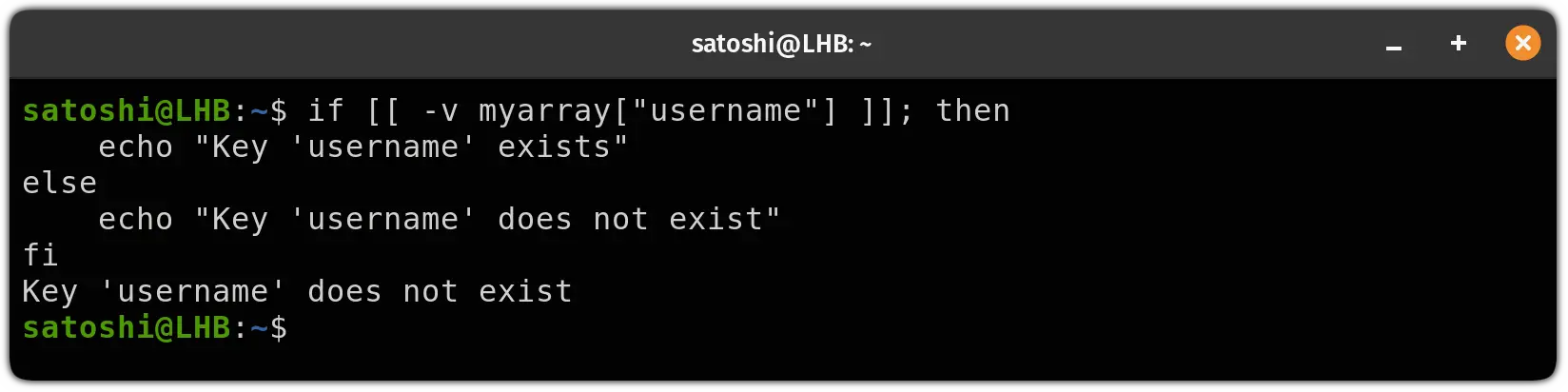
8. Clear Associative array
If you want to remove specific keys from the associative array, then you can use the unset command along with a key you want to remove:
unset my_array["key1"]
For example, if I want to remove the email
key from the myarray
array, then I will use the following:
unset myarray["email"]
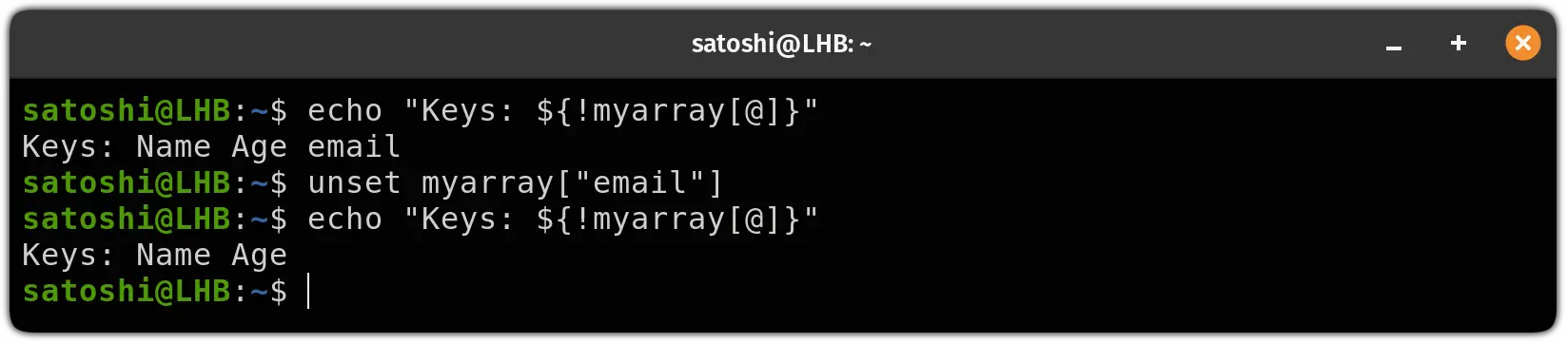
9. Delete the Associative array
If you want to delete the associative array, all you have to do is use the unset command along with the array name as shown here:
unset my_array
For example, if I want to delete the myarray
array, then I would use the following:
unset myarray
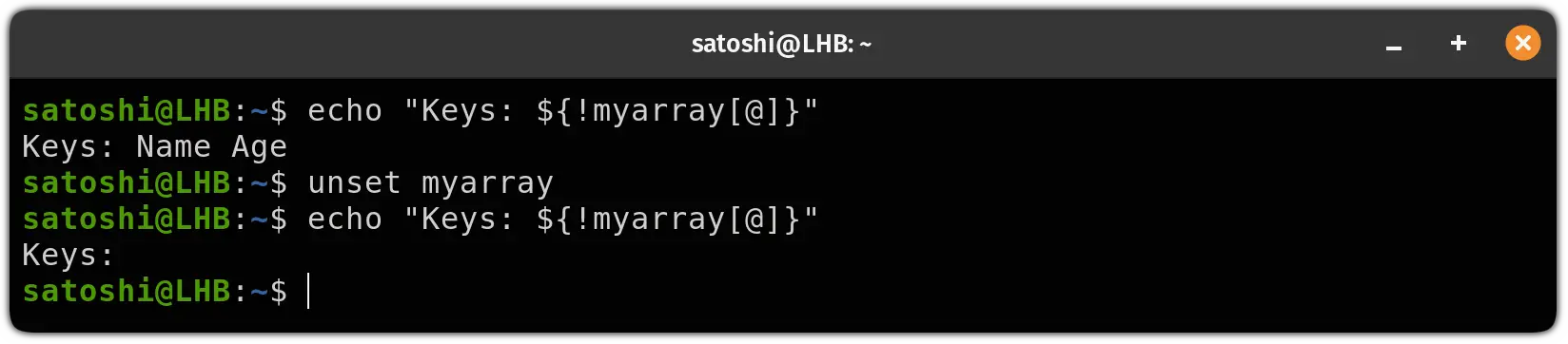
Wrapping Up…
In this tutorial, I went through the basics of the associative array with multiple examples. I hope you will find this guide helpful.
If you have any questions or suggestions, leave us a comment.