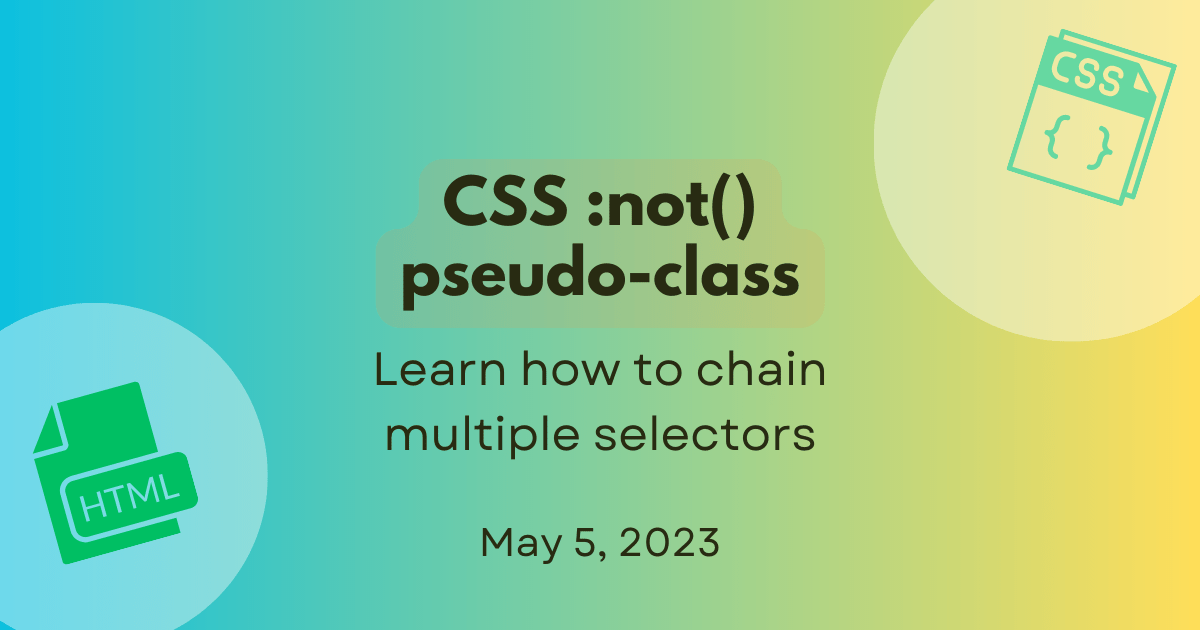
In CSS, when you want to apply the same style to multiple elements, you generally use a comma-separated selector list to group the selectors. This is illustrated below:
h1,
h2,
h3,
h4,
h5,
h6 { font-family: helvetica;
}
Similarly, you can group several :not()
declarations in a comma-separated list of selectors to apply the specified styles only to excluded matching elements. The result is a style that applies to any element excluded by the :not()
selector.
Let’s say we have a form where we want to style only the text fields that are editable. In the form in the example below, the field Employed
is a menu option field, the Employment Date
field has the type date
, and the field Country
has the attribute readonly
. These are the fields that we want to exclude from styling and the ones we can target by using the :not()
pseudo-class.
This is the HTML code for the above form where you can spot the attributes and type:
<form> <label for="name" class="name">Name:</label> <input type="text" id="name" name="name" value="Enter your name" /> <label for="emp" class="emp">Employed:</label> <select name="emp" id="emp" disabled> <option selected>No</option> <option>Yes</option> </select> <label for="empDate">Employment Date:</label> <input type="date" name="empDate" id="empDate" /> <label for="country">Country:</label> <input type="text" name="country" id="country" value="United States" readonly /> <label for="resume">Resume:</label> <input type="file" name="resume" id="resume" />
</form>
The desired styling can be achieved by using the :not()
pseudo-class as shown in the CSS below. The lightyellow
background color applies to all <input>
elements that do not have the disabled
attribute or the readonly
attribute or the type date
. The result is the selection and styling of the fields Name
, Employed
, and Resume
. Notice that even though the field Employed
has the attribute disabled
, it is not styled because it is a <select>
element.
input:not([disabled], [readonly], [type="date"]) { background-color: lightyellow; color: red;
}
This is how :not()
chains the multiple selectors.