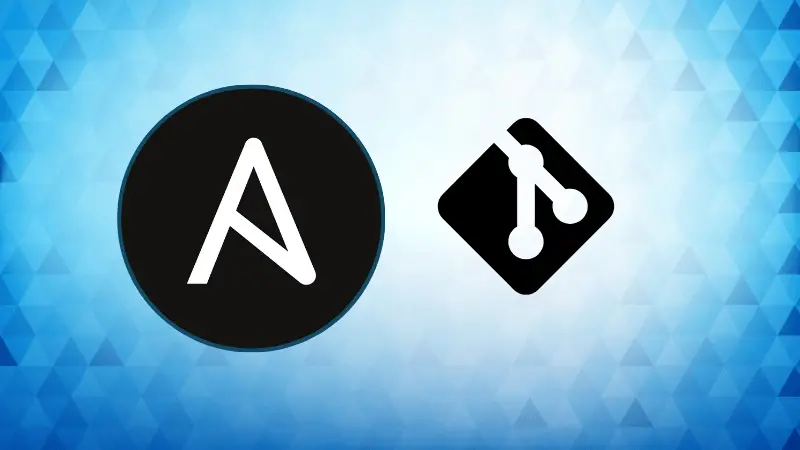
Ansible Git module allows you to easily manage Git repositories directly from your Ansible playbooks. It helps clone, fetch, and pull changes from Git repositories without manually running Git commands on remote hosts. This is especially useful for managing application code or configuration files stored in Git.
In this guide, we’ll explore how to use the Ansible Git module with practical examples.
Basic syntax of the Ansible Git Module
Before diving into the examples, let’s look at the basic syntax of the Git module in Ansible:
- name: Manage Git repository
ansible.builtin.git:
repo: <repository_url>
dest: <destination_directory>
version: <branch_or_tag>
force: yes/no
Here is the explanation:
repo
: The URL of the Git repository.dest
: The destination directory on the remote host.version
: The branch, tag, or commit hash to checkout.force
: Whether to forcefully clone or overwrite changes.
Example 1: Cloning a public Git repository
In this scenario, we`ll use the Ansible Git module to clone a public Git repository onto a remote server. This is useful when you want to deploy code or configuration files stored in a public GitHub repository.
Here’s an example playbook:
---
- name: Clone a public Git repository
hosts: all
tasks:
- name: Clone the repository
ansible.builtin.git:
repo: "https://github.com/ansible/ansible-examples.git"
dest: "/opt/ansible-examples"
version: "main"
This playbook defines a task that clones the Ansible examples repository from GitHub to the /opt/ansible-examples
directory on the remote server. We specify the branch to clone (main branch), which is the default branch in many GitHub repositories.
Example 2: Cloning a private Git repository using SSH
When working with private Git repositories, authentication is required. The Ansible Git module supports SSH-based authentication, making it secure for managing private repositories.
Here is an example:
---
- name: Clone a private Git repository using SSH
hosts: all
tasks:
- name: Ensure SSH key is present
copy:
src: "~/.ssh/id_rsa"
dest: "~/.ssh/id_rsa"
mode: 0600
- name: Clone the private repository
ansible.builtin.git:
repo: "[email protected]:yourusername/private-repo.git"
dest: "/opt/private-repo"
version: "main"
key_file: "~/.ssh/id_rsa"
In this playbook:
- We first copy the SSH private key to the remote host using the copy module. This ensures the key is available for authentication.
- We then use the Ansible Git module to clone the private repository using SSH. The
key_file
parameter specifies the private key for secure access.
Example 3: Pulling updates from an existing repository
Often, you may need to update an already cloned repository with the latest changes from the remote repository. The Ansible Git module can handle this with the update
parameter.
Here is the playbook:
---
- name: Pull updates from Git repository
hosts: all
tasks:
- name: Pull latest changes
ansible.builtin.git:
repo: "https://github.com/ansible/ansible-examples.git"
dest: "/opt/ansible-examples"
update: yes
This playbook updates the existing Git repository in /opt/ansible-examples
by pulling the latest changes. The update: yes
option ensures that the playbook fetches new commits without re-cloning the repository.
Example 4: Checkout a specific branch
In many scenarios, you may want to clone a specific branch of the repository, such as a development or feature branch. This can be done using the version
parameter.
Here is an example playbook:
---
- name: Clone and checkout a specific branch
hosts: all
tasks:
- name: Checkout the "develop" branch
ansible.builtin.git:
repo: "https://github.com/ansible/ansible-examples.git"
dest: "/opt/ansible-examples"
version: "develop"
force: yes
This playbook clones the repository and checks out the develop branch. By setting force: yes, we ensure any existing changes are overwritten if needed.
Git tags are used to mark specific points in a repository’s history. The Ansible Git module allows you to clone a repository at a specific tag.
Here is the playbook:
---
- name: Checkout a specific Git tag
hosts: all
tasks:
- name: Clone the repository at tag v1.0.0
ansible.builtin.git:
repo: "https://github.com/ansible/ansible-examples.git"
dest: "/opt/ansible-examples"
version: "v1.0.0"
This playbook clones the repository and checks out the tag v1.0.0
, ensuring the specified version of the code is deployed.
Conclusion
The Ansible Git module provides a convenient and automated way to interact with Git repositories. It simplifies tasks like cloning, updating, and managing different branches or versions, making it an essential tool for DevOps workflows.
By using these examples, you can integrate Git operations into your Ansible playbooks, ensuring a smooth and reliable deployment process.